C# String Format – formatting Strings in 2024
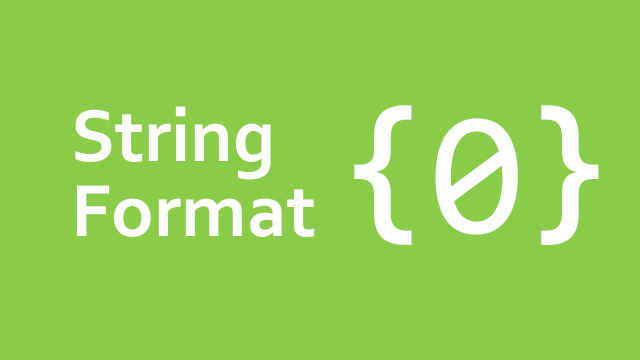
Inhaltsverzeichnis
- 1 Formatting Strings in C#
- 2 How to format a String in C#?
- 3 Formatting a String by manual concatenation
- 4 How to format a String in C# „the old way“?
- 5 How to do a C# String Format with interpolation?
- 6 How to format numbers and currencies?
- 7 How to format DateTimes or more complex objects?
- 8 Wrapping up
- 9 Related posts
Formatting Strings in C#
Using a C# String format to improve your displayed strings? No problem! Today is your lucky day, because this post will basically cover everything about formatting different things to readable strings in C#. No matter if you want to format some numbers, dates or even more complex things like objects. You will also learn more about which variants actually exist to do the formatting.
How to format a String in C#?
As mentioned above, there can be pretty different situations where you would want nicely formatted strings. Imagine having an invoice, where the value would be like „14“. At first you could say: „Well, fourteen of what?“ and usually you would like it to have two decimal points. But even things like displaying a list of persons inside a listbox will touch the string formatting topic.
The different approaches of formatting strings
Before we dive any deeper into the specific functions and helpers themselves, let’s first take a look at our possibilities in total. When it comes to formatting strings in C#, there are basically like 3 possibilities:
- concatenating strings manually – ew
- using a function of the string class – oldschool
- making use of the new string interpolation feature – yay!
If you don’t know what the term „concatenating“ a string in the context of programming means, no worries, I’ll start from scratch here. As the word itself already suggests, it basically means: „Take one string on the one hand, take another one on the other and glue them together forming a brandnew one“.
I think the easiest example for this will be the one in the next section, which covers concatenating two name parts into a full name.
Formatting a String by manual concatenation
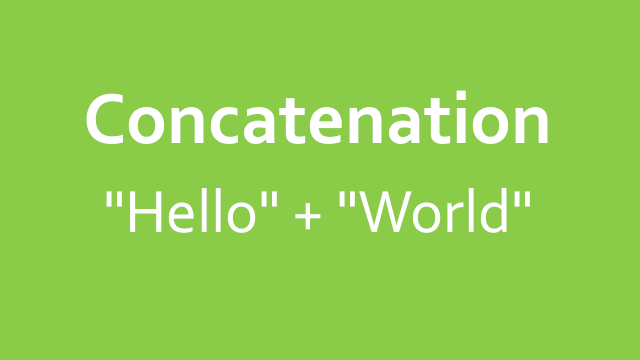
Look at the first method of formatting a string from above, we can see the basic concatenation. As already explained, this will form a brandnew string by concatenating multiple ones. Thankfully, you aren’t limitied to a specific count of strings here.
Let’s now take a look at the first little example of creating the mentioned full name string from name parts. We will create two basic variables for this in the first step, then we will combine them.
string firstName = "John"; string lastName = "Doe";
After creating those variables, we can now concatenate them into a complete new string (yup, totally new). Keep in mind that C# strings are immutable and the common operators and functions will always create a new string.
string fullName = firstName + " " + lastName;
You can now say, that this was the actual most basic formatting of a string you could do, but what if it has to be a bit more complicated? What if you are in the actual need of giving a user the change to specify his own format style? Let’s look at this in the next section of this post.
How to format a String in C# „the old way“?
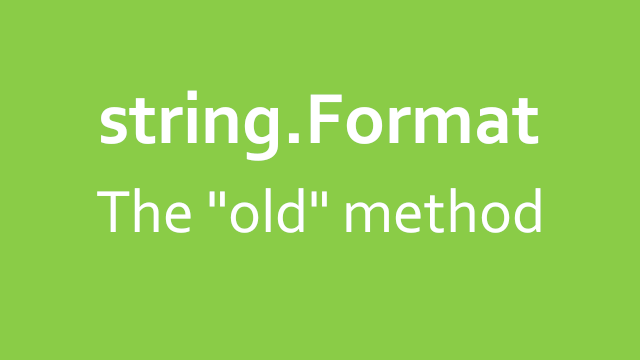
The example from the section above was pretty common and there basically is no customization chance for the user. If someone uses your program and wants to like change the way this input data is ordered, he would have to swap the first and the last name. I mean sure, sometimes it isn’t necessary/wanted, but just sayin‘!
Now let’s look at a little helper function from the NET frameworks string class, which is simply called „Format“. As the name already suggests it helps with – you guessed it – formatting strings. We will now use this function to replicate the same full name string from above.
To be able to do this, we should take a look at the function parameters first. There are different overloads, but we will focus the one having a string as the first parameter and an object parameter array as the second parameter. The first parameter wants to have some kind of formatting „formula“ to do the actual formatting. The second one wants a list of objects to actually put them in the specified places. This is done by using curly braces containing the specific index of the corresponding object.
Dynamically formatting a String
We can pass any objects (currently being two strings) by separating each one through a comma. In the next example, I will pass the two name parts. Our first name variable will then have the index 0 and the last name will be at index 1.
string firstName = "John"; string lastName= "Doe"; string fullName = string.Format("{0} {1}", firstName, lastName);
Now you could actually let your user input some kind of format of his desire (if needed). In the next example I just switched out the order of names as mentioned above. This will create a new string with the contents: „Doe John“ instead of „John Doe“.
// take this from like a TextBox, etc. string userWantedFormat = "{1} {0}"; string firstName = "John"; string lastName= "Doe"; string fullName = string.Format(format, firstName, lastName);
For sure you could also control those passed in names by adding and removing (even omitting) items to a list dynamically. Then you could also pass that list as a parameter, as the function is able to work with a list of any kind of objects as well.
How to do a C# String Format with interpolation?
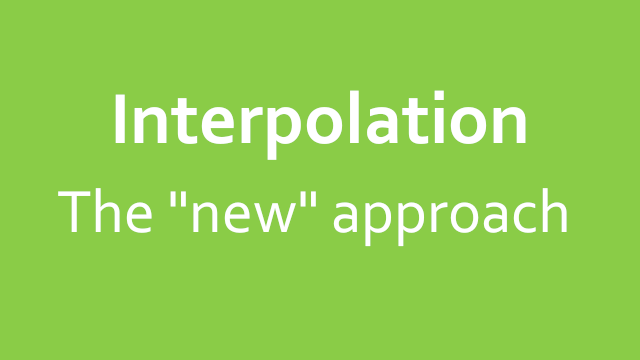
I pretty much love the approach of formatting strings in C# the „modern“ way, called „string interpolation“. This way, you can basically put the strings you want at the position of your desire – directly! Let’s now construct the above example by using the string interpolation technique.
Next to specifying the exact position, it’s pretty much easier to actually know what to put where. In the above example we could only say like „put string with index 0 here or there“, but what if the actual index changed? Will you still remember the correct position?
Here’s the example from above, but now with string interpolation. For this, we need to put a dollar sign in front of the string itself, then we can just put in those variable names by surrounding them with curly braces. In my opinion, this is much easier and clearer than all previous examples.
Like this, we can also forget about those stupid opening and closing tags of strings. With the omittance of the no longer needed „+“-signs, it’s even easier and less verbose.
string firstName = "John"; string lastName = "Doe"; string fullName = $"{firstName} {lastName}";
How to format numbers and currencies?
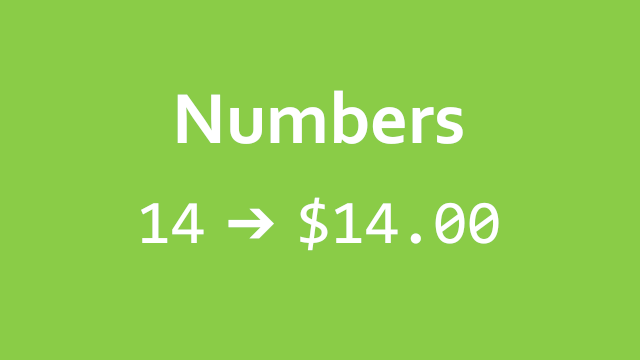
After only working on the basic first and last name example, we will go a step further right now. I mean, formatting strings to strings isn’t that hard at all, but let’s see, how it would look with more complex types. The first more complex (but still easy) type is a usual number, just like the invoice sum from the top of this post (being 14).
Usually – at least for me in germany here – you display the total sum of an invoice with two decimals. Next to those decimal points, you also use for example the Euro sign. Let’s try to use the basic representation of that number 14 displayed in the image above.
To do so, we can use one of the shown methods mentioned above:
- manual formatting
- string class method called format
- interpolating a string
Formatting with „ToString“ object base function
First, I will provide an example of doing this „manually“, by using the typical „ToString“ function being inherited from the base class object. We will use the overload, where we can specify a format as string and the format will be „N2“. This stands for format a number „into“ a string, but with two decimal points.
decimal totalNet = 14.95M; string displayValue = totalNet.ToString("N2"); // will result in displayValue being "14.95" in an en-US system setup
Using string.format or interpolation
The same results from above can be achieved with the same functionality from further above. I will use both other techniques here, as they are pretty much equal.
decimal totalNet = 14.95M; // using string.Format string displayValue = string.Format(totalNet, "{0:N2}"); // using string interpolation string displayValue = $"{totalNet:N2}";
Specifying a particular culture / language / locale
As you can see in my comment, you will get some value for „displayValue“ like „14.95“. Keep in mind, that this will be dependent on your language setting. You could also tell him, to use like the german language setting with another parameter called „FormatProvider“.
// dont forget the import! // using System.Globalization; // use either this CultureInfo deDeCulture = new CultureInfo("de-DE"); // or this CultureInfo enUsCulture = new CultureInfo("en-US"); decimal totalNet = 14.95M; // provided as second parameter here string displayValue = totalNet.ToString("N2", deDeCulture); // and look at the changed displayValue variable en-US = 14.95, de-DE = 14,95
To use string interpolation with a culture, you could create a „FormattableString“ and use this instead of a normal one.
decimal totalNet = 14.95M; // this magically creates a FormattableString! FormattableString displayValue = $"The total price is {totalNet:N2}."; CultureInfo enUsCulture = new CultureInfo("en-US"); string message = displayValue.ToString(enUsCulture ); // en-US = 14.95, de-DE = 14,95
Setting the culture globally, not for each call
In the next step, you could make it even „easier“ – depending on the use case – by setting this „CultureInfo“ more globally. Like this, you would override the system specific default setting by just providing another one. Take a look at the following snippet:
// dont forget to import // using System.Threading; CultureInfo deDeCulture = new CultureInfo("de-DE"); CultureInfo enUsCulture = new CultureInfo("en-US"); // overriding the system default to a specific value Thread.CurrentThread.CurrentCulture = enUsCulture;
All subsequent calls to formatting specific functions like „ToString“ will now use the „enUsCulture“ as default (overriding the system defaults).
Ouputting currency-formatted strings
After talking about the usual number style formatting, we can now try to display a simple currency aware number. This works pretty much the same by just changing one single letter. I changed „N2“ (which stands for number with 2 decimals) to „C2“ (which means currency with 2 decimals).
// take a look at the previous examples for string.format and manual things as well FormattableString displayValue = $"The total price is {totalNet:C2}."; CultureInfo enUsCulture = new CultureInfo("en-US"); string message = displayValue.ToString(enUsCulture );
How to format DateTimes or more complex objects?
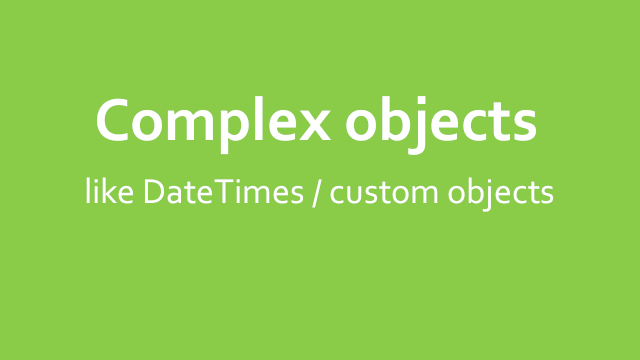
In the next section we will take a closer look at formatting more comples objects instead of those simple numbers and stuff. I’m for example talking about the „DateTime“ and other (maybe) custom classes you made yourself.
What if you have this customer which can be a private or a business customer and you want to use a C# String Format to display „him“? Are you going to display his business name, or his private first and last name? How do interpolate a string using this information?
Formatting DateTimes
Let’s first do some examples formatting an instance of the C# DateTime class. I will stay at using the string interpolation, but for sure, you can use the other techniques from above as well. After displaying a simple date string, we will also go ahead with some month-ish and time-ish strings.
// using the current date and time for example DateTime now = DateTime.Now; // displaying a typical german date - 30.03.2023 for example string displayValue = $"{now:dd.MM.yyyy}"; // displaying typical english date - care for escaping the backslashes, you could also // omit the double backslashes and use the normal @ prefix like @$ string displayValue = $"{now:MM\\dd\\yyyy}"; // working with a time string displayTimeValue = @$"{now:HH:mm}";
Formatting custom objects like a customer class instance
In this last section we will take a look at formatting our custom made customer class. For sure, this will be a pretty simple class with like only a few properties – for displaying purposes. We will create two „types“ of customers (which I would normally do otherwise, but now..): Business customers and private customers. The business customer will only have its company set, the private one will have a first and a last name.
public class Customer { public string Company { get; set; } public string FirstName { get; set; } public string LastName { get; set; } public Customer(string company) { Company = company; } public Customer(string firstName, string lastName) { FirstName = firstName; LastName = lastName; } }
We could then create a private and a business customer like this:
Customer privateCustomer = new Customer("John", "Doe"); Customer businessCustomer = new Customer("Doe Max Profit ltd.");
If you now try to format them like this, you will get some gibberish like „Customer: ProjectName.ClassName“. This is basically how objects without overriding of the „ToString“ function will look alike.
// will contain like Customer: ProjectName.Customer string text = $"Customer: {privateCustomer}";
So as mentioned as above, we only need to override the „ToString“ function to make it easier for us. For sure, you could also just display some other properties/values. In this example, I will just prioritize the company name and if not provided, I will return the full name.
public class Customer { // ... // the the other stuff from above // ... public override ToString() { if (string.IsNullOrEmpty(Company)) { return Company; } return $"{FirstName} {LastName}"; } }
Now take a look of the results of the following code lines:
Customer privateCustomer = new Customer("John", "Doe"); Customer businessCustomer = new Customer("Doe Max Profit ltd."); // will contain Customer: John Doe string text = $"Customer: {privateCustomer}"; // will contain Customer: Doe Max Profit ltd. string text = $"Customer: {businessCustomer}";
As seen in the comments, we will now be able to see some different and better looking results.
Wrapping up
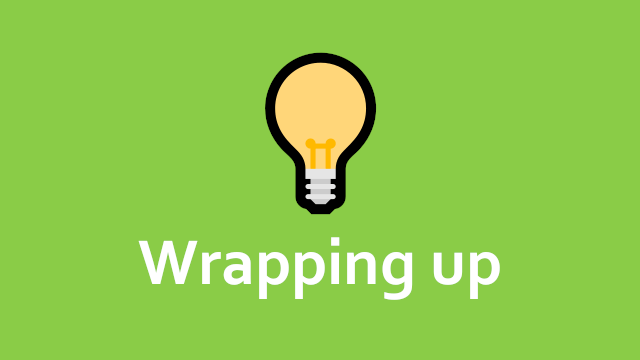
So at the end of this post, we take a quick look back of what we’ve talked (or I’ve written..) about. Formatting different things into readable strings is an often occuring problem in programming. In C#, you can basically use three different approaches: Manual concatenation, using the „Format“ helper method of the string class or the newer string interpolation. Providing an instance of the „CultureInfo“ class can help with localization either on an „per function“ basis, but globally as well. I would personally recommend staying with the interpolation approach nowadays, because is much easier to write and read.