rskibbe.I18n.Ini – An INI file based translation sub-package
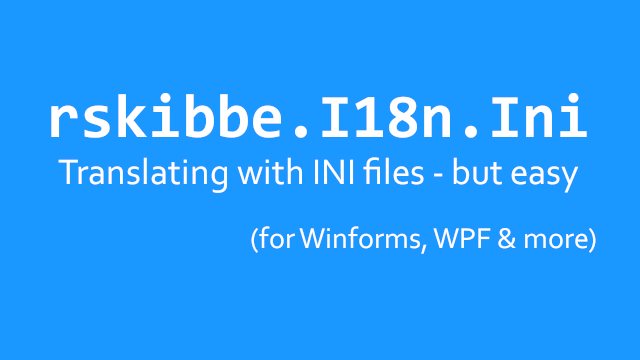
Inhaltsverzeichnis
What is rskibbe.I18n.Ini?
rskibbe.I18n.Ini is a sub-package for the rskibbe.I18n helping you by providing basic implementations for INI file based translations. Creating multilanguage / internationalized apps is easier than ever, just install the packages and start translating your Windows Forms, WPF and Console apps.
At first, we are going to take a look on how to get started. As already mentioned, this package here, is only a sub-thing for the main package, so keep in mind, that it depends on the main one. As you can probably imagine by the name, this package is designed for INI file based translation.
How to get started?
To start right away, you can use the NuGet Package Manager from your Visual Studio Tools. If you prefer the more „dev-thingy“ installation style, you can also use the NuGet console. Search for „rskibbe.I18n“ and you will a few corresponding packages. For this example, you will need the two listed below:
// install the base package first Install-Package rskibbe.I18n // then execute this as well, to install the sub-package Install-Package rskibbe.I18n.Ini
Namespaces
As soon as you are done installing these two packages successfully, you can now continue by importing the most important namespaces. With these, you will have the fluent builder interface available and you are ready to go. Keep in mind, that I won’t go to deep into the documentation of the base package. Therefore, I would recommend going to the base package documentation, if you need more details about it.
using rskibbe.I18n.Models; using rskibbe.I18n.Ini;
Imports rskibbe.I18n.Models Imports rskibbe.I18n.Ini
You can now start through with building your custom translator, fitting your indivual needs inside your Windows Forms, WPF or Console App.
Setup
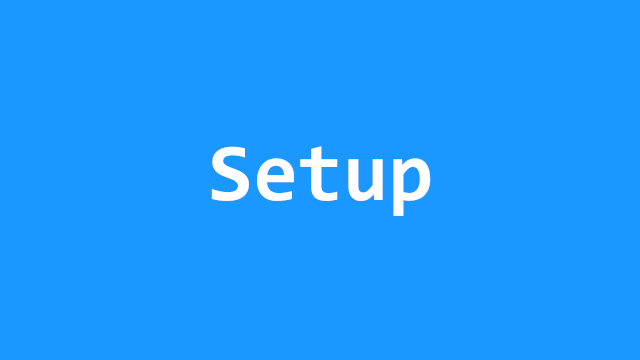
Creating the folder structure
Right inside your output directory (where the executable is, when like Debugging your project, etc.), create the following folder structure: At the root, there is the base „i18n“ folder, then there is another subfolder called „ini“ and inside of it, there is the languages.ini. You can specify and provide different languages and translations by adding them into the languages.ini and providing the corresponding translations by having an INI file for each language.
- bin
- debug
- i18n
- ini
- languages.ini
- en-US_English.ini
- de-DE_Deutsch.ini
- ini
- i18n
- debug
languages.ini
In this file, you need to specify all available languages for your application. Remember, we are using the INI format! A matching example for the structure mentioned above, could look like this:
[English] Iso=en-US Name=English [Deutsch] Iso=de-DE Name=Deutsch
I specified the german and english language over here. Each one with a name and an iso „property“. The section header content doesn’t really matter – more or less. Empty lines get ignored.
Translation files
In the next step, we are going to create the matching translation files – in our case: „en-US_English.ini“ and „de-DE_Deutsch.ini„. The pattern for those files is pretty simple, just mention the correct iso code (this needs to match your languages file). Then you should append an underscore with the specific language name.
For our example from above, the translation files could look like these:
en-US_English.ini
[en-US_English] key1=Actions greeting=Hello!
de-DE_Deutsch.ini
[de-DE_Deutsch] key1=Aktionen greeting=Hallo!
The section headers get ignored, but I think it feels right to provide them anyways, because this is just how a normal Ini would look like, right? But feel free to omit them. The corresponding translation key would be „key1“, which would result in „Actions“ or „Aktionen“ depending on the language.
Code
Please don’t forget to take a look at the basic setup of the base package. Here’s just a quick example of the installed INI implementations of ILanguagesLoader & ITranslationTablesLoader. Setting up the translator should be done as early as possible.
Further, we will tell the „TranslatorBuilder“ to literally use the installed helpers. Then, it will know how to load the corresponding languages and even more, how to load the matching translations.
// don't forget the imports somewhere above.. using rskibbe.I18n.Models; using rskibbe.I18n.Ini; // create and save the instance for later, DI, etc. var translator = Translator.Builder .WithLanguagesLoader<IniLanguagesLoader>() .WithTranslationTableLoader<IniTranslationTableLoader>() .Build() .StoreInstance();
' don't forget the imports somewhere above.. Imports rskibbe.I18n.Models Imports rskibbe.I18n.Ini ' create and save the instance for later, DI, etc. Dim translator = Translator.Builder _ .WithLanguagesLoader(Of IniLanguagesLoader)() _ .WithTranslationTableLoader(Of IniTranslationTableLoader)() _ .Build() _ .StoreInstance()
Full Code example
Here’s how a complete example could look like. Please don’t forget to install the packages, import the namespaces and check the project namespace as well.
using rskibbe.I18n.Models; using rskibbe.I18n.Ini; namespace <YourProjectName>; public partial class Form1 : Form { public Form1() { var translator = Translator.Builder .WithLanguagesLoader<IniLanguagesLoader>() .WithTranslationTableLoader<IniTranslationTableLoader>() .Build() .StoreInstance(); InitializeComponent(); Load += Form1_Load; } private async void Form1_Load(object? sender, EventArgs e) { await Translator.Instance.LoadLanguagesAsync(); // after the languages have been loaded, the translator // set the language automatically, if WithLanguage("en-US") // has been used during initialization // if not, you could just trigger the initial language // change/set now // await Translator.Instance.ChangeLanguageAsync("en-US"); // you could now get a specific translation by // (keep in mind, that on using WithLanguage, you // would actually need to like listen to the initial // LanguageChanged event, to get the translation) ITranslation translation = Translator.Instance.Translate("key1"); // translation.Value -> Actions in en-US, Aktionen in de-DE } }
Imports rskibbe.I18n.Models Imports rskibbe.I18n.Ini Namespace <YourProjectName> Public Partial Class Form1 Inherits Form Public Sub New() Dim translator = Translator.Builder _ .WithLanguagesLoader(Of IniLanguagesLoader)() _ .WithTranslationTableLoader(Of IniTranslationTableLoader)() _ .Build() _ .StoreInstance() InitializeComponent() End Sub Private Async Sub Form1_Load(ByVal sender As Object?, ByVal e As EventArgs) Handles MyBase.Load Await Translator.Instance.LoadLanguagesAsync() Dim translation As ITranslation = Translator.Instance.Translate("key1") End Sub End Class End Namespace
Take a look at the base package for more information like getting the available languages, changing the language, etc.